Today i am talking about tooltip, First of all i introduce you , what is tooltip? When our mouse cursor move over the object then appear a rectangle shape with text this things known as tooltip. When we work with particular item then easy set the tooltip on it. But when we work on collection then we put some ideas on it. Like first to access each item from the collection then apply tooltip on each accessed item. Today, i want to give a simple example on it. I have a database table with three columns, see below:
Database table(country table)
CREATE TABLE [dbo].[country] (
[CountryId] INT IDENTITY (1, 1) NOT NULL,
[CountryName] NVARCHAR (MAX) NULL,
[Countryimage] NVARCHAR (MAX) NULL,
PRIMARY KEY CLUSTERED ([CountryId] ASC)
);
First to add some item in the table after then you have to access items. If you want to add items in the table by the help visual studio server explorer then do the following.
Right click on your table name --> Select show table data
Source Code
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default9.aspx.cs" Inherits="Default9" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:DropDownList ID="DropDownList1" runat="server" Height="26px" OnDataBound="DropDownList1_DataBound" Width="142px" DataSourceID="SqlDataSource1" DataTextField="CountryName" DataValueField="CountryId"></asp:DropDownList>
<asp:SqlDataSource ID="SqlDataSource1" runat="server" ConnectionString="<%$ ConnectionStrings:ConnectionString %>" SelectCommand="SELECT * FROM [country]"></asp:SqlDataSource>
</div>
</form>
</body>
</html>
How to design above mentioned code. The answer is :Bind the DropdownList with the SqlDataSource also SqlDataSource Control connect with database table. The above mentioned code automatically generated by the visual studio.
Code Behind Code
protected void DropDownList1_DataBound(object sender, EventArgs e)
{
DropDownList ddl = sender as DropDownList;
if (ddl != null)
{
foreach (ListItem li in ddl.Items)
{
li.Attributes["title"] = li.Text;
}
}
}
After bind the Dropdownlist you have to access each item from the list by using DataBound event. Use foreach loop to access all item one by one from the list also apply tooltip on each item by the help of Attribute property.
ToolTip on specific DropdownList item in asp.net c#
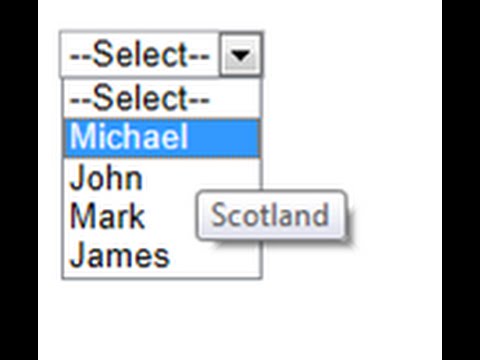