After binding the DataGrid in wpf. You can insert some item in the database table using WPF TextBox. So first of all add one label , TextBox and one button control in the grid container. Insert data in the table on button click so add button click event on button.
<Grid>
<Grid Margin="0,0,10,203">
<Label Content="Enter Name Here:" /> <TextBox Name="text1" Text="" Margin="112,4,228,0" Height="27" VerticalAlignment="Top" />
<Button Content="Add Data" Margin="76,46,310,22" Click="Button_Click" />
</Grid>
<DataGrid Name="g1" AutoGenerateColumns="False" Margin="110,121,137,57">
<DataGrid.Columns>
<DataGridTextColumn Binding="{Binding Id}" Header="Id" Width="100"/>
<DataGridTextColumn Binding="{Binding Name}" Header="Name" Width="100"/>
</DataGrid.Columns>
</DataGrid>
</Grid>
</Window>
If you want to show inserted item then need to bind DataGrid along with other control. Check my previous article.
C# Code
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Windows;
namespace WpfApplication2
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
binddatagrid();
}
private void binddatagrid()
{
SqlConnection con = new SqlConnection();
con.ConnectionString = ConfigurationManager.ConnectionStrings["connstudent"].ConnectionString;
con.Open();
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "select * from [Students]";
cmd.Connection = con;
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataTable dt = new DataTable("Students");
da.Fill(dt);
g1.ItemsSource = dt.DefaultView;
con.Close();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
SqlConnection con = new SqlConnection();
con.ConnectionString = ConfigurationManager.ConnectionStrings["connstudent"].ConnectionString;
con.Open();
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "Insert into [Students](Name)values(@nm)";
cmd.Parameters.AddWithValue("@nm", text1.Text);
cmd.Connection = con;
int a = cmd.ExecuteNonQuery();
if(a==1)
{
MessageBox.Show("Data add Sucessfully");
binddatagrid();
}
}
}
}
Above mentioned code is similar to all application like asp.net, windows form etc. Apply sql insert query with the SqlCommand class and execute NonQuery() method to insert the item in the database table.
How to insert data into database table in WPF application
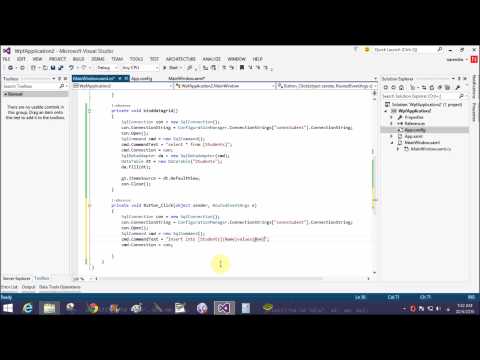